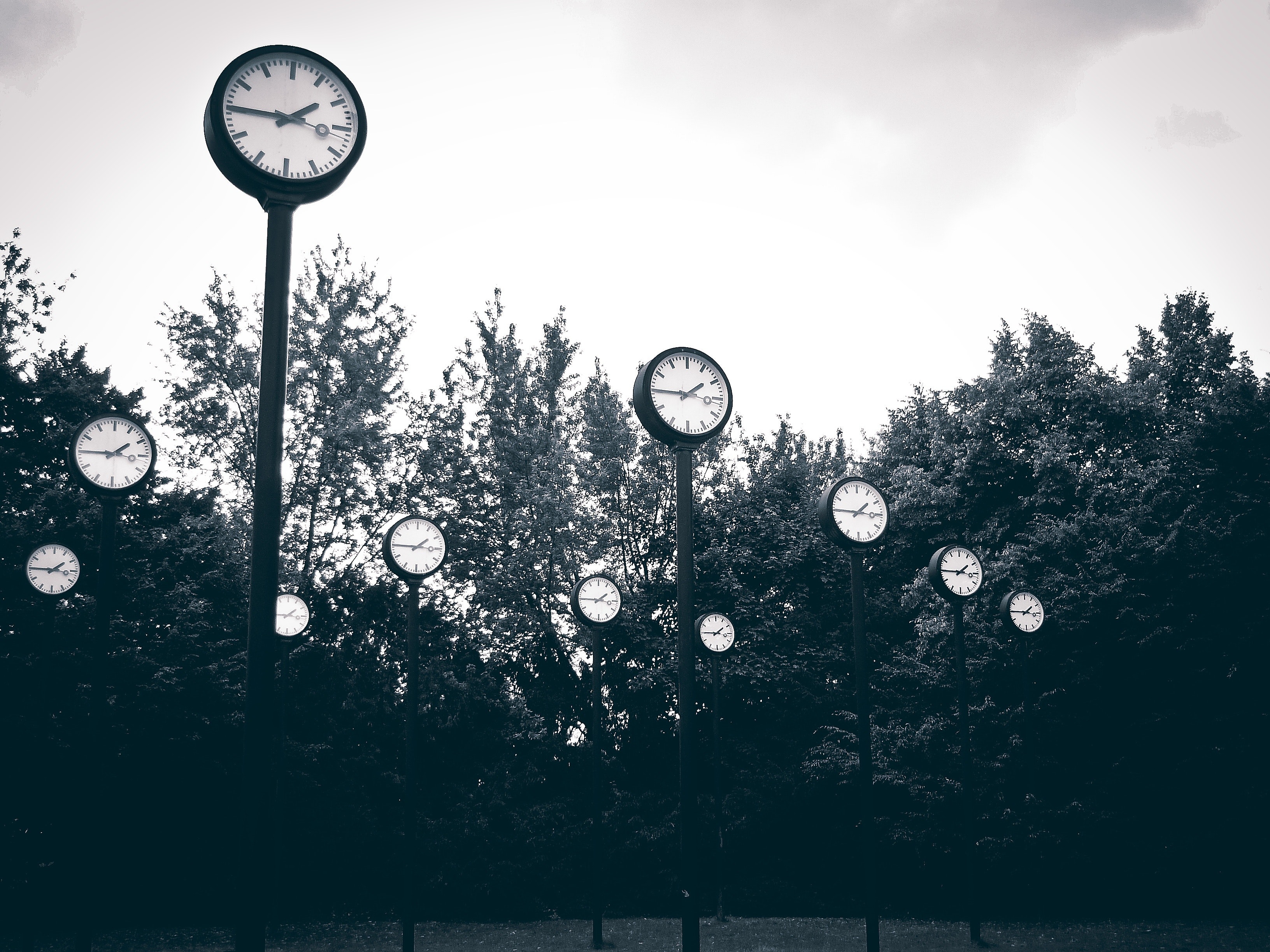
In this post we are going to develop a simple cron job using CronDock
After signing up the CronDock web portal will provide you with an "API key" which can be used to call the CronDock RESTful API. Note that at this moment you can only communicate with CronDock through the API. Using the API key you can call the CronDock via different tools such as "curl", python libraries such as "requests" or tools such as postman .
The CronDock API end point is https://api.crondock.com/
.
Keep in mind that in all your API calls to CronDock API endpoint, you should add your "API key" in
a Header called "Authorization" and you need to add "Api-Key
" at its begining. So for instance in
"curl" you will have something like (replace XXXXX
with your "API key"):
curl -X GET -H "Content-Type: application/json" -H "Authorization: Api-Key XXXXX" https://api.crondock.com/task/Or in python:
import requests headers = { 'Content-Type': 'application/json', 'Authorization': 'Api-Key XXXXX', } response = requests.get('https://api.crondock.com/task/', headers=headers)
To create a simple cron job using CronDock, you should start with creating a "task".
task can have two different type
s: simple
and cron
.
A simple
task is a job that runs only once right after being created.
This can be used to programmatically run any docker image on CronDock from your code.
A cron
task is a scheduled task that runs a docker frequently on CronDock based on
the schedule
you provide for it. In this post we are going to start with a simple
task
first and then we are going to move forward and create a cron
task.
To create a simple
you need to submit a POST
request to
/task/
endpoint and pass some parameters as part of the payload.
The parameters are as follow:
name
: a name for your job, note that this name will get updated with a suffix by the
system to avoid having the same name for different runs.image
: path to the container image you want to run, note that with a free account you can only
run public images and you need a basic or enterprise account for running images from private repositories.args
: the argument to pass to the container, note that the arguments should be provided as
a list of string values.type
: the type of the task which can be either simple
or cron
,
note that simple
tasks run only once after being created and cron
tasks run frequently based on the provided
schedule
schedule
: the schedule to run the cron job. This should be a string that defines the schedule
of the task and should follow the cron format (you can read more about cron format here: https://crontab.guru/).
Note that this only can be used in tasks with type
set as cron
.curl
from docker hub
(curlimages/curl:latest
) for our examples here. You can learn more about this image here.
In this example we want to read the https://curl.haxx.se
website as a simple task. So we submit a POST
request to /task/
endpoint like this:
curl -X POST --url http://api.crondock.com/task/ \ -H "Content-Type: application/json" \ -H "Authorization: Api-Key XXXX" \ -d '{ "name": "curl-simple", "image": "curlimages/curl:latest", "type": "simple", "args": ["-L", "-v", "https://curl.haxx.se"] }'The API will response with something like this:
{ "status": "OK", "data": { "id": 21, "created_at": "2022-06-23T21:42:36.746984Z", "name": "curl-simple-u48msb", "image": "curlimages/curl:latest", "args": ["-L", "-v", "https://curl.haxx.se"], "type": "simple", "schedule": "", "user": 10 } }What is important from above is
"id": 21
part which basically
tells us what is the id of the new task we just created. You can read more about the API request for adding "task" in
the document.
So now let's take a look at the results. In order to do that we need to submite a GET
request to
/task/
endpoint. We can specify the task we want to see by passing the
task id
like this: /task/21/
where 21
is the task id here from above.
If we don't specify the task id
the api will response with all the existing tasks
on the account.
curl -X GET --url http://localhost:9000/task/21/ \ -H "Content-Type: application/json" \ -H "Authorization: Api-Key XXXX"The API will response with something like this:
{ "count":1, "next":null, "previous":null, "results":[{ "id":21, "created_at":"2022-06-23T21:42:36.746984Z", "name":"curl-simple-u48msb", "image":"curlimages/curl:latest", "args":["-L","-v","https://curl.haxx.se"], "type":"simple", "schedule":"", "user":10, "results":[{ "status":{ "start_time":"2022-06-23 21:43:32+00:00", "phase":"Succeeded", "message":null, "init_container_statuses":null, "ephemeral_container_statuses":null }, "metadata":{ "name":"curl-simple-u48msb--1-855ns" } }] } ]}
The start_time
field shows when the task started running (UTC time) and
the phase
field shows the status of the task which in this case is Succeeded
.
If you need to see the logs of the tasks you can pass logs=true
as a parameter in the url like this:
curl -X GET --url http://localhost:9000/task/21/?log=true \ -H "Content-Type: application/json" \ -H "Authorization: Api-Key XXXX"And the result will be something like this:
{ "count":1, "next":null, "previous":null, "results":[{ "id":21, "created_at":"2022-06-23T21:42:36.746984Z", "name":"curl-simple-u48msb", "image":"curlimages/curl:latest", "args":["-L","-v","https://curl.haxx.se"], "type":"simple", "schedule":"", "user":10, "results":[{ "status":{ "start_time":"2022-06-23 21:43:32+00:00", "phase":"Succeeded", "message":null, "init_container_statuses":null, "ephemeral_container_statuses":null }, "metadata":{ "name":"curl-simple-u48msb--1-855ns" }, "log": " % Total % Received % Xferd Average Speed Time Time Time Current\n Dload Upload Total Spent Left Speed\n\r 0 0 0 0 0 0 0 0 --:--:-- --:--:-- --:--:-- 0* Trying 146.75.38.49:443...\n* ...< !DOCTYPE HTML PUBLIC \"-//W3C//DTD HTML 4.01 Transitional//EN\" \"http://www.w3.org/TR/html4/loose.dtd\"\n >< html> < head> < title>curl/title> ... < /body>\n < /html>\n " }] } ]}
In The log
field you can see all the logs from running that task.
So this was a simple
task, next let's create a cron
task.
In order to do that in the POST
request you need to change the type
to
cron
and also set a schedule
for the task. In this example we are going to use
30 17 * * *
as the schedule meaning that the task is going to run every day at 17:30 UTC time.
So the API request will be like this:
curl -X POST --url http://api.crondock.com/task/ \ -H "Content-Type: application/json" \ -H "Authorization: Api-Key XXXX" \ -d '{ "name": "curl-cron", "image": "curlimages/curl:latest", "type": "cron", "schedule": "30 17 * * *" "args": ["-L", "-v", "https://curl.haxx.se"] }'And the API will response with something like this:
{ "status": "OK", "data": { "id": 23, "created_at": "2022-06-23T23:00:07.363589Z", "name": "curl-cron-kwzmfs", "image": "curlimages/curl:latest", "args": ["-L", "-v", "https://curl.haxx.se"], "type": "cron", "schedule": "30 17 * * *", "user": 10 } }
And on CronDock now this task will run every day 17:30 UTC time on CronDock!
This article shows how you can create a cron job on CronDock. Please let me know if you have any question at support@crondock.com
I also have a good document which provides more detailed information on all the requests you can make to CronDock API.